Interaction between GridObjects#
In this Jupyter Notebook, the magic functions of the gridObject are explained. These are operations like adding two GridObjects together. Instead of utilizing functions that could look like new_dem = topo.add(dem1, dem2)
, this package utilizes magic functions. The same addition will therefor look like this: new_dem = dem1 + dem2
.
The following operations are possible using GridObjects:
Equality Check:
==
Inequality Check:
!=
Greater Than:
>
Less Than:
<
Greater Than or Equal To:
>=
Less Than or Equal To:
<=
Addition:
+
Subtraction:
-
Multiplication:
*
Division:
/
Logical AND:
&
Logical OR:
|
Logical XOR:
^
Length:
len()
Iteration:
for i in dem:
Item Access:
y = dem[x]
Item Assignment:
dem[x] = y
Array Representation: Passing the GridObject in places where a numpy.ndarray is expected
String Representation:
print(dem)
[1]:
import topotoolbox as topo
import numpy as np
Iterating through a GridObject:#
[2]:
dem = topo.gen_random_bool(rows=4, columns=4)
# looping through a GridObject
for i in dem:
print(i)
# Accessing cells of an GridObject
print(f"\n{dem[2][2]}")
dem[2][2] = 2
print(f"\n{dem[2][2]}")
[0. 1. 1. 0.]
[0. 0. 1. 1.]
[0. 1. 1. 0.]
[1. 1. 1. 0.]
1.0
2.0
Comparing GridObjects#
[3]:
dem1 = topo.gen_random(rows=32, columns=32, hillsize=24)
dem2 = topo.gen_random(rows=32, columns=32, hillsize=32)
# Some comparisons between the two generated GridObjects
topo.show(dem1, dem2, dem1 > dem2, dem1 == dem2)
---------------------------------------------------------------------------
AttributeError Traceback (most recent call last)
Cell In[3], line 5
2 dem2 = topo.gen_random(rows=32, columns=32, hillsize=32)
4 # Some comparisons between the two generated GridObjects
----> 5 topo.show(dem1, dem2, dem1 > dem2, dem1 == dem2)
File ~/.local/lib/python3.10/site-packages/topotoolbox/utils.py:96, in show(dpi, cmap, *grid)
94 for i, dem in enumerate(grid):
95 ax = axes[i]
---> 96 im = ax.imshow(dem, cmap=cmap)
97 ax.set_title(dem.name)
98 fig.colorbar(im, ax=ax, orientation='vertical')
AttributeError: 'numpy.ndarray' object has no attribute 'imshow'
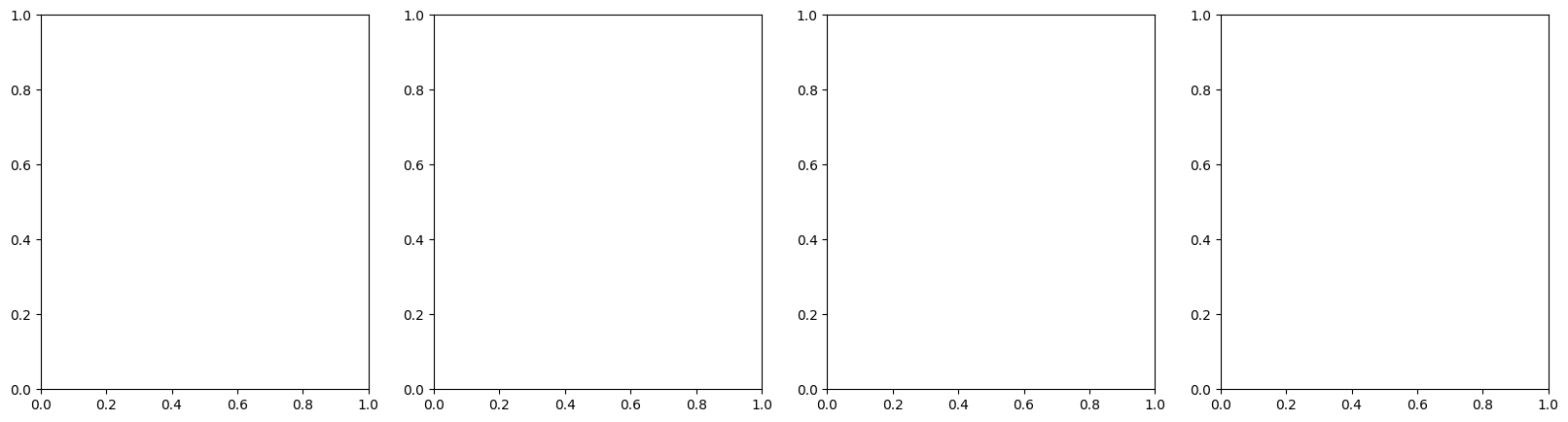
Using AND, OR as well as XOR#
[4]:
dem1 = topo.gen_random_bool()
dem2 = topo.gen_random_bool()
topo.show(dem1, dem2, dem1 & dem2, dem1 | dem2, dem1 ^ dem2)
---------------------------------------------------------------------------
AttributeError Traceback (most recent call last)
Cell In[4], line 5
1 dem1 = topo.gen_random_bool()
2 dem2 = topo.gen_random_bool()
----> 5 topo.show(dem1, dem2, dem1 & dem2, dem1 | dem2, dem1 ^ dem2)
File ~/.local/lib/python3.10/site-packages/topotoolbox/utils.py:96, in show(dpi, cmap, *grid)
94 for i, dem in enumerate(grid):
95 ax = axes[i]
---> 96 im = ax.imshow(dem, cmap=cmap)
97 ax.set_title(dem.name)
98 fig.colorbar(im, ax=ax, orientation='vertical')
AttributeError: 'numpy.ndarray' object has no attribute 'imshow'
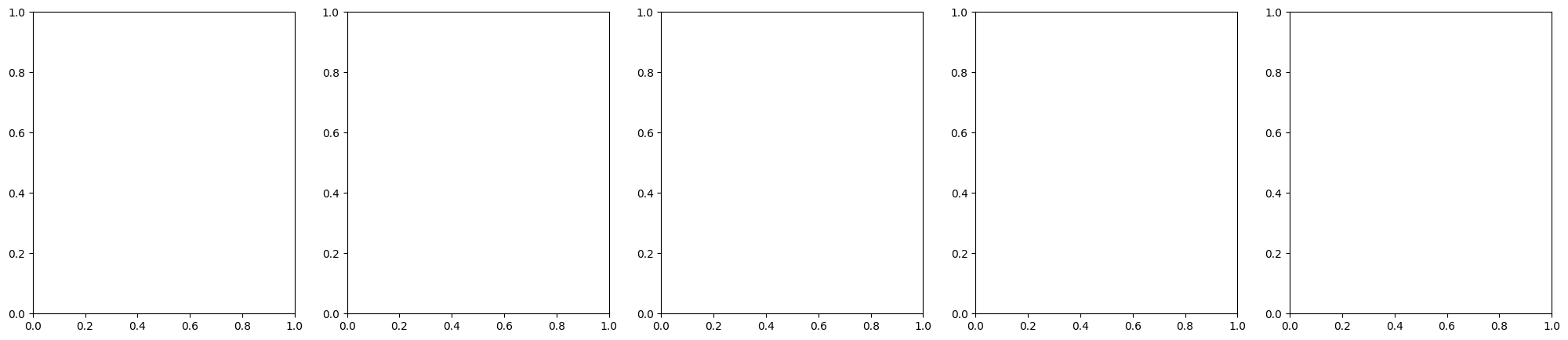
[5]:
dem1 = topo.gen_random(rows=32, columns=32, seed=128, name='dem1')
dem2 = topo.gen_random(rows=32, columns=32, seed=84, name='dem2')
# Adding two GridObject together and multiplying dem1 by 2. Notice the colorbar
topo.show(dem1, dem2, dem1 + dem2, dem1*2)
---------------------------------------------------------------------------
AttributeError Traceback (most recent call last)
Cell In[5], line 5
2 dem2 = topo.gen_random(rows=32, columns=32, seed=84, name='dem2')
4 # Adding two GridObject together and multiplying dem1 by 2. Notice the colorbar
----> 5 topo.show(dem1, dem2, dem1 + dem2, dem1*2)
File ~/.local/lib/python3.10/site-packages/topotoolbox/utils.py:96, in show(dpi, cmap, *grid)
94 for i, dem in enumerate(grid):
95 ax = axes[i]
---> 96 im = ax.imshow(dem, cmap=cmap)
97 ax.set_title(dem.name)
98 fig.colorbar(im, ax=ax, orientation='vertical')
AttributeError: 'numpy.ndarray' object has no attribute 'imshow'
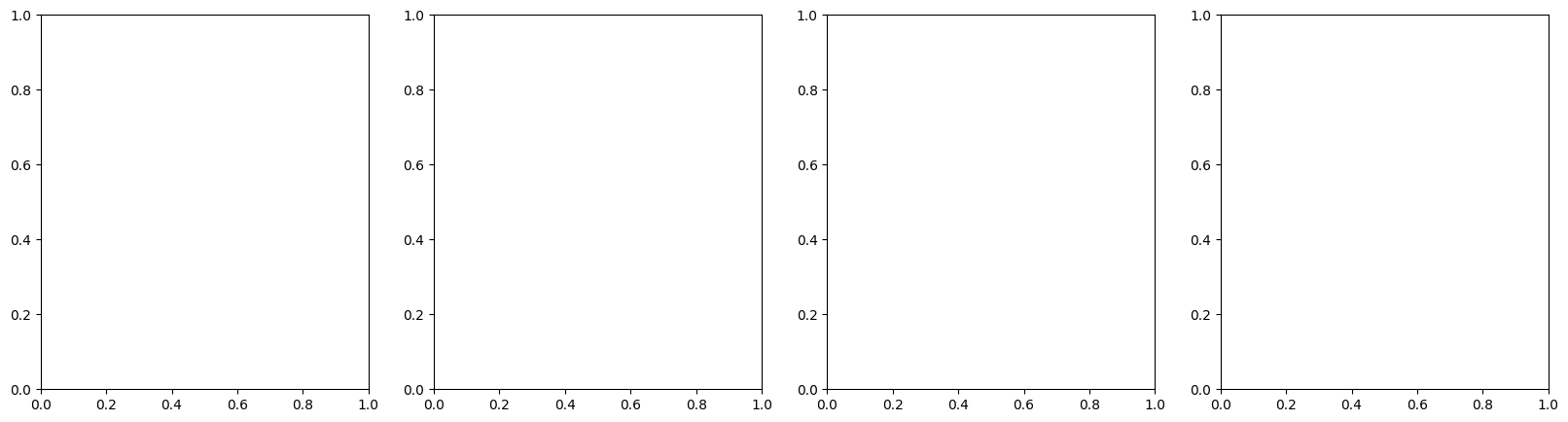